跟冒泡排序有点相似,冒泡排序是符合条件直接进行交换,而选择排序则是将索引记录下,一轮比较完成后再进行交换。
找最小元素
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| #include <iostream> #include <vector> using namespace std;
void SelectionSort(vector<int>& vec); void Print(const vector<int>& vec);
int main() { vector<int> vec = { 49, 38, 65, 97, 76, 13, 27, 49 }; cout << "before sort:" << endl; Print(vec); cout << "sort:" << endl; SelectionSort(vec); cout << "after sort:" << endl; Print(vec); }
void SelectionSort(vector<int>& vec) { int length = vec.size(); int minIndex; for( int i = 0; i < length - 1; i++ ) { minIndex = i; for( int j = i + 1; j < length; j++ ) { if( vec[j] < vec[minIndex] ) { minIndex = j; } }
if( minIndex != i ) { swap(vec[i], vec[minIndex]); }
Print(vec); } }
void Print(const vector<int>& vec) { for( int v : vec ) { cout << v << " "; } cout << endl; }
|
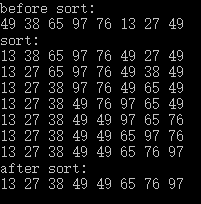
找最大元素
跟上者的过程相反,代码看起来会没上者的简洁。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| #include <iostream> #include <vector> using namespace std;
void SelectionSort(vector<int>& vec); void Print(const vector<int>& vec);
int main() { vector<int> vec = { 49, 38, 65, 97, 76, 13, 27, 49 }; cout << "before sort:" << endl; Print(vec); cout << "sort:" << endl; SelectionSort(vec); cout << "after sort:" << endl; Print(vec); }
void SelectionSort(vector<int>& vec) { int length = vec.size(); int maxIndex; for( int i = 0; i < length - 1; i++ ) { maxIndex = length - 1 - i; for( int j = length - 1 - i - 1; j >= 0; j-- ) { if( vec[j] > vec[maxIndex] ) { maxIndex = j; } }
if( maxIndex != length - 1 - i ) { swap(vec[length - 1 - i], vec[maxIndex]); }
Print(vec); } }
void Print(const vector<int>& vec) { for( int v : vec ) { cout << v << " "; } cout << endl; }
|
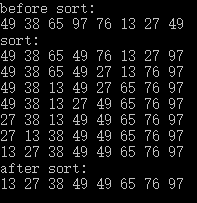
打印出来的排序过程跟上者是不同的。